Migrate Content Providers to Jetpack App Startup (Android)
If you (or any third-party library) uses Content Providers to initialize parts of your app, then you should migrate them to the Jetpack App Startup library. Each content provider adds a penalization to your startup times. So, removing these providers can help to reduce your startup performance. The Jetpack App Startup library provides a performant way to initialize components at application startup. Both library developers and app developers can use this library to streamline startup sequences and explicitly set the order of initialization.
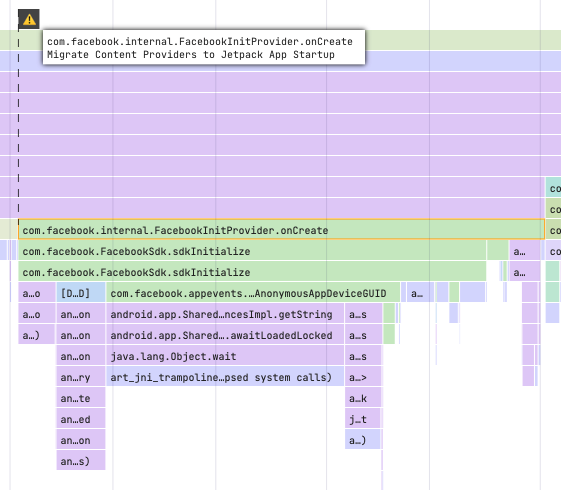
Some third-party SDKs automatically merge Content Providers int your app build by the Android build tools when doing a build with Gradle. Here are some examples of how to migrate them to the Jetpack App Startup library:
Firebase SDK
In your manifest, add an entry for FirebaseInitProvider, and make sure to use a node marker to set its tools:node attribute to the value "remove". This tells the Android build tools not to include this component in your app:
<provider
android:name=”com.google.firebase.provider.FirebaseInitProvider” android:authorities=”${applicationId}.firebaseinitprovider” tools:node=”remove” />
Because you removed FirebaseInitProvider, you’ll need to perform the same init somewhere in your app (in your own Jetpack App Startup Initializer, to ensure Analytics can measure your app correctly):
if (FirebaseApp.initializeApp(context) == null) {
Log.w(TAG, “FirebaseApp initialization unsuccessful”)
} else {
Log.i(TAG, “FirebaseApp initialization successful”)
}
More info here.
ML Kit
In your manifest, add an entry for MlKitInitProvider, and make sure to use a node marker to set its tools:node attribute to the value "remove". This tells the Android build tools not to include this component in your app:
<provider
android:name=”com.google.mlkit.common.internal.MlKitInitProvider” android:authorities=”${applicationId}.mlkitinitprovider” tools:node=”remove” />
Because you removed MlKitInitProvider, you’ll need to perform the same init somewhere in your app (in your own Jetpack App Startup Initializer, to ensure Analytics can measure your app correctly):
MlKit.initialize(context)
WorkManager
The WorkManager library uses Jetpack App Startup to automatically initialize since v2.6.0. Be sure to update your library to the latest version to improve your startup time.
Facebook SDK
In your manifest, add an entry for FacebookInitProvider, and make sure to use a node marker to set its tools:node attribute to the value "remove". This tells the Android build tools not to include this component in your app:
<provider
android:name=”com.facebook.internal.FacebookInitProvider” android:authorities="${applicationId}.FacebookInitProvider" tools:node=”remove” />
Because you removed FacebookInitProvider, you’ll need to perform the same init somewhere in your app (in your own Jetpack App Startup Initializer, to ensure Analytics can measure your app correctly):
FacebookSdk.sdkInitialize(context)
Sentry SDK
In your manifest, add an entry for SentryInitProvider, and make sure to use a node marker to set its tools:node attribute to the value "remove". This tells the Android build tools not to include this component in your app:
<provider
android:name=”io.sentry.android.core.SentryInitProvider” android:authorities=”${applicationId}.SentryInitProvider” tools:node=”remove” />
Because you removed SentryInitProvider, you’ll need to perform the same init somewhere in your app (in your own Jetpack App Startup Initializer, to ensure Analytics can measure your app correctly):
SentryAndroid.init(context, if (BuildConfig.DEBUG) NoOpLogger.getInstance() else SystemOutLogger())
Updated 3 months ago