Firebase API Exposed (Android)
By default, the Android API Key created by Firebase can expose sensitive remote config data for your app. Emerge scans your app and warns you if this security issue is detected.
The issue
When you create a Firebase account, some API Keys are automatically generated on the Google Cloud Console. You can see them on https://console.cloud.google.com/apis/credentials.
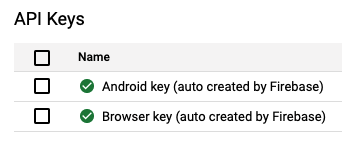
If you click on Android key (auto created by Firebase), you will see that by default it has no Application restrictions:
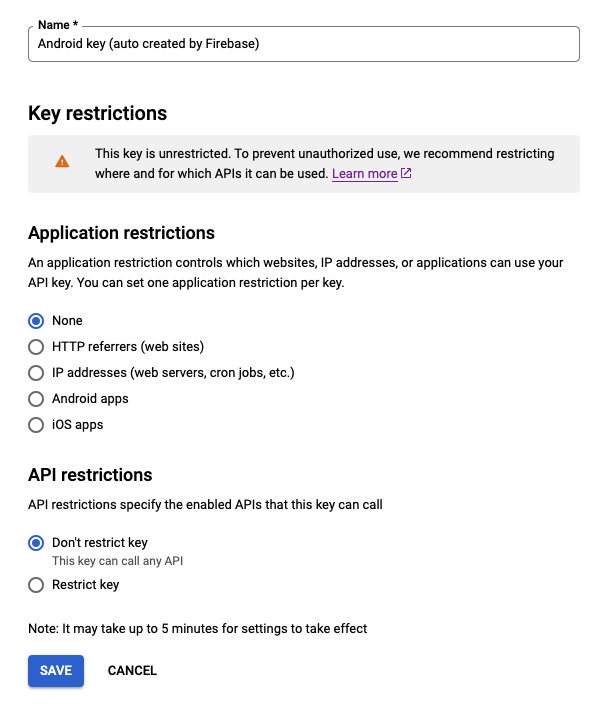
This means that anyone could invoke some read-only Google Cloud/Firebase APIs if they have the proper keys.
When you integrate Firebase on your android app, you are required to copy the google-services.json file on your project. This file contains your project id and the API key, and it will be part of your APK/AAB.
So, anyone could get your app keys from your app and invoke a read-only Firebase API. The steps to do that are pretty simple:
- Open the Android APK/AAB with Android Studio APK Analyzer (Build -> Analyze APK)
- Open the strings resources and find the Google API Key (
google_api_key
), Firebase Project ID (project_id
), and the Google App ID (google_app_id
) - Execute the following request, replacing the
PROJECT_ID
,API_KEY
, andAPP_ID
values with the ones you found in step 2.
curl -v -X POST "https://firebaseremoteconfig.googleapis.com/v1/projects/{PROJECT_ID}/namespaces/firebase:fetch?key={API_KEY}" -H "Content-Type: application/json" --data '{"appId": "{APP_ID}", "appInstanceId": "PROD"}'
- If the vulnerability is present, you will get a JSON with all your Remote Config Parameters and their values on the response:
{
"entries": {
"key1": "value1"
},
"state": "UPDATE",
"templateVersion": "1"
}
Why is this important?
Exposing the remote config information associated with your app can allow users to uncover sensitive secrets, experimentation information, feature flags, and any information you might store in Firebase Remote Config.
While Firebase recommends against storing any sensitive data in Remote Config, exposing any remote config is a poor security practice and is an easy vulnerability to expose at scale.
The fix
You can’t avoid having those keys exposed on the APK/AAB, because Firebase SDK needs them. But you can restrict access to the API.
Go to https://console.cloud.google.com/apis/credentials and select the Android key (auto created by Firebase), then you will see an Application Restrictions section. You need to pick the Android Apps option and add an entry for each application id / Keystore SHA1 of your app.
For example:
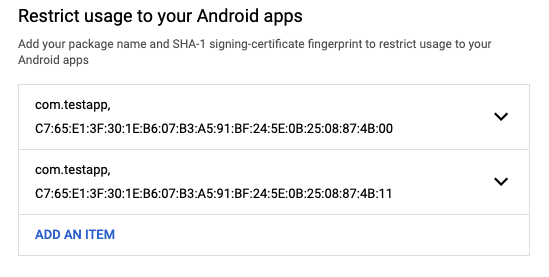
Remember to take into account the SHA1 for the following Keystores:
- Your debug Keystore (the Keystore you use during development)
- Your upload Keystore (the Keystore you use to upload the APK/AAB to Google Play)
- Your Google Play Keystore (the Keystore generated by Google Play if you have Play App Signing enabled)
- Your Firebase App Distribution Keystore
After doing that, only the application with the proper signature will be able to access the Firebase APIs. You will get the following error if you try to request the API without the correct signature:
{
"error": {
"code": 403,
"message": "Requests from this Android client application \u003cempty\u003e are blocked.",
"status": "PERMISSION_DENIED",
"details": [
{
"@type": "type.googleapis.com/google.rpc.ErrorInfo",
"reason": "API_KEY_ANDROID_APP_BLOCKED",
"domain": "googleapis.com",
"metadata": {
"service": "firebaseremoteconfig.googleapis.com",
"consumer": "projects/123456789"
}
}
]
}
}
Updated almost 2 years ago